Input
POST https://gateway.appypie.com/sdxl_lightning/getImage/v1/getSDXLImage HTTP/1.1 Content-Type: application/json Cache-Control: no-cache { "prompt": "Image of Bird" }
import urllib.request, json try: url = "https://gateway.appypie.com/sdxl_lightning/getImage/v1/getSDXLImage" hdr ={ # Request headers 'Content-Type': 'application/json', 'Cache-Control': 'no-cache', } # Request body data = data = json.dumps(data) req = urllib.request.Request(url, headers=hdr, data = bytes(data.encode("utf-8"))) req.get_method = lambda: 'POST' response = urllib.request.urlopen(req) print(response.getcode()) print(response.read()) except Exception as e: print(e)
// Request body const body = { "prompt": "Image of Bird" }; fetch('https://gateway.appypie.com/sdxl_lightning/getImage/v1/getSDXLImage', { method: 'POST', body: JSON.stringify(body), // Request headers headers: { 'Content-Type': 'application/json', 'Cache-Control': 'no-cache',} }) .then(response => { console.log(response.status); console.log(response.text()); }) .catch(err => console.error(err));
curl -v -X POST "https://gateway.appypie.com/sdxl_lightning/getImage/v1/getSDXLImage" -H "Content-Type: application/json" -H "Cache-Control: no-cache" --data-raw "{ \"prompt\": \"Image of Bird\" }"
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import java.util.HashMap; import java.util.Map; import java.io.UnsupportedEncodingException; import java.io.DataInputStream; import java.io.InputStream; import java.io.FileInputStream; public class HelloWorld { public static void main(String[] args) { try { String urlString = "https://gateway.appypie.com/sdxl_lightning/getImage/v1/getSDXLImage"; URL url = new URL(urlString); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); //Request headers connection.setRequestProperty("Content-Type", "application/json"); connection.setRequestProperty("Cache-Control", "no-cache"); connection.setRequestMethod("POST"); // Request body connection.setDoOutput(true); connection .getOutputStream() .write( "{ \"prompt\": \"Image of Bird\" }".getBytes() ); int status = connection.getResponseCode(); System.out.println(status); BufferedReader in = new BufferedReader( new InputStreamReader(connection.getInputStream()) ); String inputLine; StringBuffer content = new StringBuffer(); while ((inputLine = in.readLine()) != null) { content.append(inputLine); } in.close(); System.out.println(content); connection.disconnect(); } catch (Exception ex) { System.out.print("exception:" + ex.getMessage()); } } }
$url = "https://gateway.appypie.com/sdxl_lightning/getImage/v1/getSDXLImage"; $curl = curl_init($url); curl_setopt($curl, CURLOPT_CUSTOMREQUEST, "POST"); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); # Request headers $headers = array( 'Content-Type: application/json', 'Cache-Control: no-cache',); curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); # Request body $request_body = '{ "prompt": "Image of Bird" }'; curl_setopt($curl, CURLOPT_POSTFIELDS, $request_body); $resp = curl_exec($curl); curl_close($curl); var_dump($resp);
Output
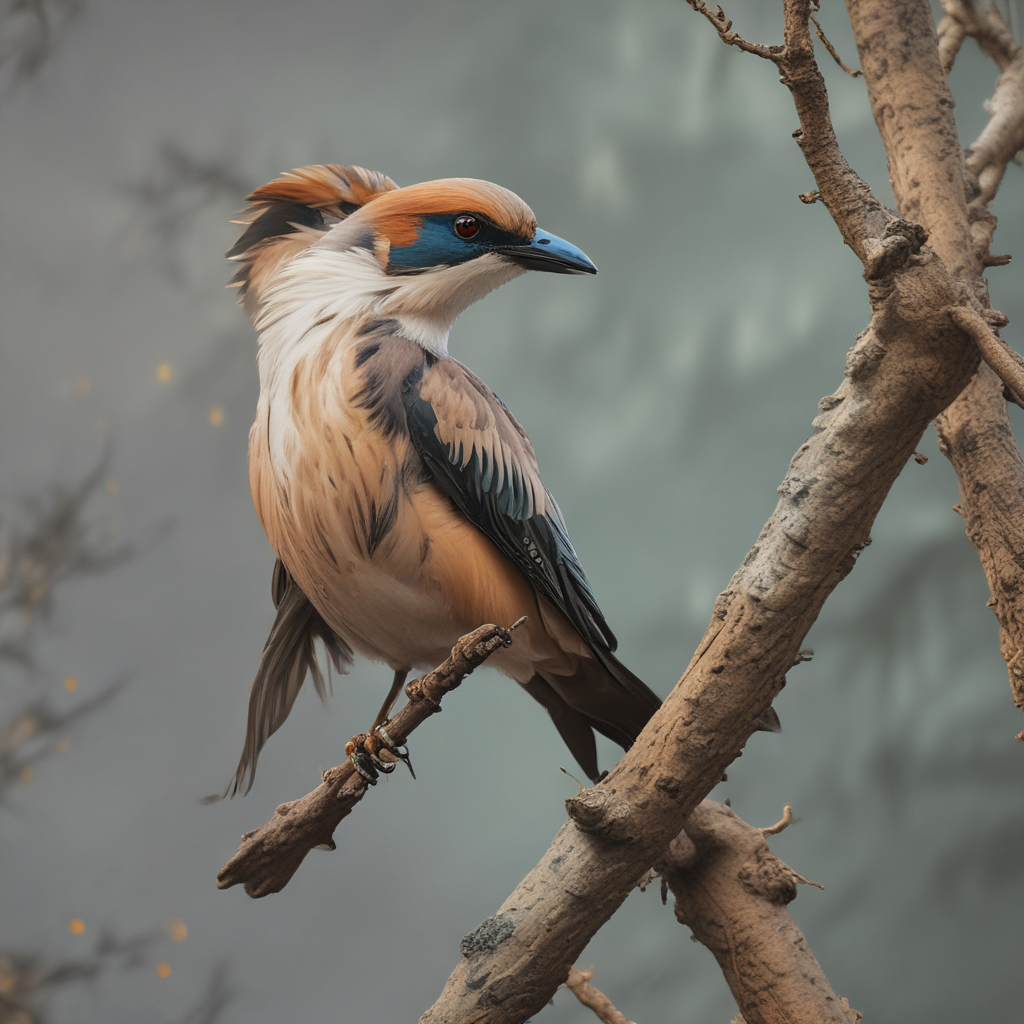
-
SDXL Lightning API Documentation
-
API Parameters
The API POST-https://gateway.appypie.com/sdxl_lightning/getImage/v1/getSDXLImage takes the following parameters:
prompt
string, required
negative_prompt
string, optional
response_format
JavaScript Object Notation (JSON)
size
string, optional, default: 1024x1024
Integration and Implementation
To utilize the Stable Diffusion XL API, developers need to send POST requests to the designated endpoint, ensuring they include the correct headers and request body. The request body should contain the text prompt along with any additional parameters such as negative prompts and image size. Comprehensive documentation themes are provided to enhance the documentation experience, guiding users through the integration process.
Base URL
https://gateway.appypie.com/sdxl_lightning/getImage/v1/getSDXLImage
Endpoints
POST /getImage
This endpoint generates images based on the provided text prompts.
Request
- URL: https://gateway.appypie.com/sdxl_lightning/getImage/v1/getSDXLImage
- Method: POST
- Headers:
- Content-Type: application/json
- Cache-Control: no-cache
- Ocp-Apim-Subscription-Key: {subscription_key}
- Body:
JSON
{ "prompt": "Change to a cat" }
- HTTP Status Codes:
- 200 OK: The request was successful, and the generated image is included in the response body.
- 400 Bad Request: The request needed to be corrected or include some arguments.
- 401 Unauthorized: The API key provided in the header is invalid.
- 500 Internal Server Error: An error occurred on the server while processing the request.
- Sample Response:
JSON
{ "status": 200, "content-type": "image/png", "content-length": "size_in_bytes", "image_data": "base64_encoded_image_data" }
- Error Field Contract:
- code: An integer that indicates the HTTP status code (e.g., 400, 401, 500).
- message: A clear and concise description of what the error is about.
- traceId A unique identifier that can be used to trace the request in case of issues.
- AI Model: Refers to the underlying machine learning model used to interpret the text prompts and generate corresponding images.
- Changelog: Document detailing any updates, bug fixes, or improvements made to the API in each version.
- Digital Content Creation: Content creators can harness the capabilities of the stable diffusion XL lighting API to generate high-quality images for various digital platforms such as social media, websites, and blogs. Whether it's creating visually appealing graphics, generating custom illustrations, or enhancing photographs, the API provides versatile tools for content creation.
- E-commerce Visuals: E-commerce platforms can leverage the SDXL lighting API to generate captivating product images, visualize customizations, and create compelling visual content for product listings. By incorporating generated images, businesses can enhance product presentation, leading to increased user engagement and sales.
- Virtual Try-Ons: Retailers in the fashion and beauty industry can seamlessly integrate the stable diffusion XL lighting API to offer immersive virtual try-on experiences to their customers. By generating realistic images of customers wearing different clothing or makeup products, businesses can enable shoppers to make informed purchase decisions online.
- Personalized Marketing: Marketers can leverage the SDXL lighting API to craft personalized visual content tailored to individual preferences and demographics. By analyzing user data and generating relevant images, businesses can deliver targeted marketing campaigns that resonate with their audience, leading to higher conversion rates and customer satisfaction.
- Artificial Intelligence Chatbots: AI-powered chatbots can elevate user interactions by integrating stable diffusion XL lighting API-generated images into their responses. Whether it's providing visual answers to user queries or engaging users with personalized visual content, the API enhances the conversational experience and improves user engagement.
- Educational Resources: Educational platforms and e-learning tools can enrich their offerings by incorporating visually engaging educational materials created using the stable diffusion XL lighting API. From interactive diagrams to illustrations and infographics, educators can enhance learning experiences and improve content comprehension for students across various subjects and disciplines.
- Dynamic Text-to-Image Generation: With the SDXL Lightning API's dynamic text-to-image generation, users can effortlessly breathe life into textual descriptions, transforming them into stunning visual compositions. Harnessing cutting-edge machine learning techniques, the API interprets textual prompts with unparalleled precision, crafting images that vividly capture the essence of the provided descriptions. This process of text generation seamlessly integrates with the API's capabilities, enabling users to specify the desired number of images to be generated in a single request.
- Customizable Image-to-Image Translation: Empowering users with unparalleled creative freedom, the API offers customizable image-to-image translation capabilities. Whether it's converting sketches into photorealistic masterpieces or altering the time of day in a scene, users can seamlessly manipulate images to suit their artistic vision, unlocking a world of creative possibilities. The image-to-image prompting feature enables users to provide images as prompts for generating new images, enhancing the flexibility and versatility of the generation process.
- Enhanced Image Refinement: Elevating image quality to sublime levels, the SDXL Lightning API boasts enhanced image refinement capabilities. Through sophisticated algorithms and advanced refinement models, users can polish and perfect their images with precision, ensuring every detail is impeccably rendered and every pixel exudes unparalleled clarity and realism. This includes the utilization of the refiner model to enhance the quality of generated images, resulting in superior image quality.
- Dynamic Image Composition: Enabling users to unleash their creativity, the SDXL Lightning API offers dynamic image composition features. From seamlessly blending different artistic styles to intelligently integrating text overlays, users can effortlessly compose visually stunning images that captivate and inspire. The API also supports the incorporation of Image URLs as input data for generating new images, facilitating dynamic content generation and adaptation to specific user needs or preferences.
- Real-Time Image Synthesis: With real-time image synthesis capabilities, users can experience instantaneous feedback and preview of generated images. Whether it's fine-tuning parameters or exploring creative variations, the API empowers users to iterate rapidly and experiment freely, accelerating the creative process and fostering innovation. The SDXL Turbo feature further enhances the speed and efficiency of the generation process, ensuring rapid production of high-quality images at scale.
- Advanced Error Handling: Equipped with robust error-handling mechanisms, the API ensures seamless operation and uninterrupted workflow. From gracefully handling unexpected inputs to providing informative error messages, users can rely on the API's resilience and stability to deliver consistent performance in any scenario.
- Comprehensive Documentation and Support: Complementing its advanced features, the SDXL Lightning API offers comprehensive documentation and robust support resources. From detailed API reference guides to interactive tutorials and troubleshooting assistance, users have access to everything they need to maximize their utilization of the API and unleash their creative potential. This includes detailed documentation on Stable Diffusion SDXL 1.0, text-to-image synthesis, and the utilization of Random seed for controlling the variability and diversity of generated images.
- API Endpoints: The SDXL Lightning API provides a sharp focus on well-defined endpoints, ensuring seamless interaction for developers. With its intuitive design and robust architecture, users can leverage HTTP methods to access functionalities effortlessly.
- Request Formats: Users can submit requests to the API in structured formats like JSON, facilitating clear communication of parameters such as sharp focus, shallow depth of field, and larger UNet backbone. This ensures accurate processing and generation of images that meet specific requirements.
- Response Structures: The API delivers responses in standardized formats, emphasizing visual fidelity and clarity. Whether it's image data, metadata, or error messages related to noisy latent or visual fidelity, the response structure is meticulously crafted for easy interpretation and integration.
- Scalability and Performance: Engineered for scalability and performance, the SDXL Lightning API effortlessly handles large volumes of requests while maintaining minimal latency. With its emphasis on stability.ai and efficiency, users can rely on consistent performance even under heavy workloads.
- Security: Security measures are paramount in the SDXL Lightning API, safeguarding data integrity and confidentiality. Authentication mechanisms, encryption protocols, and access controls are in place to protect against unauthorized access, ensuring the safety of sensitive information.
- Documentation and Support: Comprehensive documentation accompanies the API, providing detailed insights into its functionalities and usage guidelines. Developers can access tutorials, code samples, and developer forums to navigate the API effectively and harness its capabilities to the fullest.
- Advanced Image Generation: With the Text to Image SDXL API, users can harness cutting-edge machine learning models and sophisticated algorithms to create stunning visuals that push the boundaries of imagination. From lifelike portraits to captivating fantasy art, the API transforms text embeddings and input images into captivating designs and compositions.
- Versatility and Flexibility: Designed to meet the diverse needs of enterprise clients and developers, the SDXL Lightning API provides a wide range of design elements and image solutions. Whether it's enhancing existing images or generating entirely new ones, the API adapts to various use cases and scenarios, offering unparalleled flexibility and customization options.
- High-Quality Outputs: Enterprise developers can rely on the SDXL Lightning API to deliver high-quality outputs that meet the rigorous standards of enterprise clients. With its emphasis on visual fidelity and precision, the API ensures that generated images are not only visually stunning but also true to the original vision, catering to the discerning needs of enterprise users.
- Efficiency and Scalability: Engineered for efficiency and scalability, the SDXL Lightning API streamlines image generation processes and can handle large volumes of requests with ease. Whether it's serving a single user or an entire enterprise client base, the API offers consistent performance and reliability, ensuring smooth operation and seamless integration into enterprise workflows.
- Ease of Integration: With its well-defined endpoints and comprehensive documentation, the SDXL Lightning API makes it easy for developers to integrate its functionalities into their applications. Whether you're building a custom image generation tool or enhancing existing products with visual elements, the API provides the tools and resources needed for seamless integration and development.
Responses
Error Handling
The Stable Diffusion XL API is equipped with robust error-handling mechanisms to ensure smooth operation. Users may encounter typical status codes during the interaction, including those associated with AI image generation models. Typical status codes encountered include:
Definitions
Overview of the SDXL Lightning Model
The SDXL Lightning API, developed by Stability AI, stands out as a premier AI image generation technology designed to offer unparalleled capabilities in creating, enhancing, and transforming images. This advanced API leverages state-of-the-art machine learning techniques, including latent diffusion models and other cutting-edge neural network architectures. At its core, the SDXL Lightning API features a suite of specialized models that cater to a wide array of applications, from digital art and graphic design to technical imaging and beyond. By integrating these powerful models, users can achieve highly realistic and detailed image outputs that align closely with their creative and practical needs.
One of the standout components of the SDXL Lightning API is its use of style transfer models. These models allow users to blend different artistic styles with image content, transforming ordinary visuals into unique artistic creations. These models are particularly useful in digital art, marketing, and media, where the fusion of styles can significantly enhance image composition. Additionally, the text-to-image models utilize advanced transformers and diffusion techniques to generate detailed images from textual descriptions, making them perfect for advertising, concept art, and automated graphic design.
The versatility of the SDXL Lightning API extends further with its super-resolution, inpainting, and image-to-image translation models. Super-resolution models enhance the resolution of low-quality images, which is crucial for improving photo quality, medical imaging, and upscaling visuals for high-resolution displays. Inpainting models fill in missing or corrupted parts of images, seamlessly restoring and editing visuals, essential for photo restoration and object removal. Lastly, image-to-image translation models facilitate the transformation of images from one domain to another, such as converting sketches into photorealistic images or altering the time of day in a scene. This wide array of capabilities underscores the API’s adaptability, making it an invaluable tool for developers and artists aiming to push the boundaries of visual creativity and innovation.
For those looking to dive deeper into the functionalities, the SDXL Lightning API tutorial provides comprehensive guidance on leveraging these models effectively. The tutorial covers various aspects such as configuring the guidance_scale parameter to balance creativity and adherence to text prompts and using text-encoders to improve the alignment of output images with input descriptions. The API also supports the Stable Diffusion XL 1.0 and Stable Diffusion 1.5 models, ensuring high-resolution image synthesis and the generation of multiple images in a single request. These features make the SDXL Lightning API an essential tool for modern image generation and manipulation, offering robust solutions for a broad spectrum of creative and practical applications. The extensive documentation and the refinement model enhance usability, making it easier for users to integrate the API into their workflows and achieve superior lightning output text results.
Use Cases of SDXL Lightning API
The SDXL Lightning API unfolds a plethora of mesmerizing use cases, each brimming with creativity and innovation. Let's delve into these important applications:
Advanced Features of the SDXL Lightning API
The SDXL Lightning API introduces advanced features designed to enhance image generation like never before. Let's take a closer look at what it offers:
Technical Specifications of the Stable Diffusion Lightning API
The SDXL Lightning API, powered by Stability.AI, embodies innovation and excellence in image generation technology. With its emphasis on visual fidelity, scalability, and security, the API empowers users to create stunning visuals with ease and confidence, revolutionizing the landscape of image generation powered by Large Language Models. Let's dive into its technical intricacies:
What are the Benefits of Using SDXL Lightning API?
The SDXL Lightning API offers a plethora of benefits, catering to developers, businesses, and users with its advanced capabilities and versatile features. Let's delve into these advantages:
Top APIs for Generative AI Models
Unlock the full potential of your projects with our Generative AI APIs. from video generation APIs to image creation, text generation, animation, 3D models, prompt generation, image restoration, and code generation, we offer advanced APIs for all your generative AI needs.