Input
POST https://gateway.appypie.com/inpainting/v1/getImage HTTP/1.1 Content-Type: application/json Cache-Control: no-cache Ocp-Apim-Subscription-Key: 8338a365bd3d4092bf6e2d9fbb54e0d1 { "prompt": "Change to a lion" }
import urllib.request, json try: url = "https://gateway.appypie.com/inpainting/v1/getImage" hdr ={ # Request headers 'Content-Type': 'application/json', 'Cache-Control': 'no-cache', 'Ocp-Apim-Subscription-Key': '8338a365bd3d4092bf6e2d9fbb54e0d1', } # Request body data = data = json.dumps(data) req = urllib.request.Request(url, headers=hdr, data = bytes(data.encode("utf-8"))) req.get_method = lambda: 'POST' response = urllib.request.urlopen(req) print(response.getcode()) print(response.read()) except Exception as e: print(e)
// Request body const body = { "prompt": "Change to a lion" }; fetch('https://gateway.appypie.com/inpainting/v1/getImage', { method: 'POST', body: JSON.stringify(body), // Request headers headers: { 'Content-Type': 'application/json', 'Cache-Control': 'no-cache', 'Ocp-Apim-Subscription-Key': '8338a365bd3d4092bf6e2d9fbb54e0d1',} }) .then(response => { console.log(response.status); console.log(response.text()); }) .catch(err => console.error(err));
curl -v -X POST "https://gateway.appypie.com/inpainting/v1/getImage" -H "Content-Type: application/json" -H "Cache-Control: no-cache" -H "Ocp-Apim-Subscription-Key: 8338a365bd3d4092bf6e2d9fbb54e0d1" --data-raw "{ \"prompt\": \"Change to a lion\" }"
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import java.util.HashMap; import java.util.Map; import java.io.UnsupportedEncodingException; import java.io.DataInputStream; import java.io.InputStream; import java.io.FileInputStream; public class HelloWorld { public static void main(String[] args) { try { String urlString = "https://gateway.appypie.com/inpainting/v1/getImage"; URL url = new URL(urlString); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); //Request headers connection.setRequestProperty("Content-Type", "application/json"); connection.setRequestProperty("Cache-Control", "no-cache"); connection.setRequestProperty("Ocp-Apim-Subscription-Key", "8338a365bd3d4092bf6e2d9fbb54e0d1"); connection.setRequestMethod("POST"); // Request body connection.setDoOutput(true); connection .getOutputStream() .write( "{ \"prompt\": \"Change to a lion\" }".getBytes() ); int status = connection.getResponseCode(); System.out.println(status); BufferedReader in = new BufferedReader( new InputStreamReader(connection.getInputStream()) ); String inputLine; StringBuffer content = new StringBuffer(); while ((inputLine = in.readLine()) != null) { content.append(inputLine); } in.close(); System.out.println(content); connection.disconnect(); } catch (Exception ex) { System.out.print("exception:" + ex.getMessage()); } } }
$url = "https://gateway.appypie.com/inpainting/v1/getImage"; $curl = curl_init($url); curl_setopt($curl, CURLOPT_CUSTOMREQUEST, "POST"); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); # Request headers $headers = array( 'Content-Type: application/json', 'Cache-Control: no-cache', 'Ocp-Apim-Subscription-Key: 8338a365bd3d4092bf6e2d9fbb54e0d1',); curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); # Request body $request_body = '{ "prompt": "Change to a lion" }'; curl_setopt($curl, CURLOPT_POSTFIELDS, $request_body); $resp = curl_exec($curl); curl_close($curl); var_dump($resp);
Output
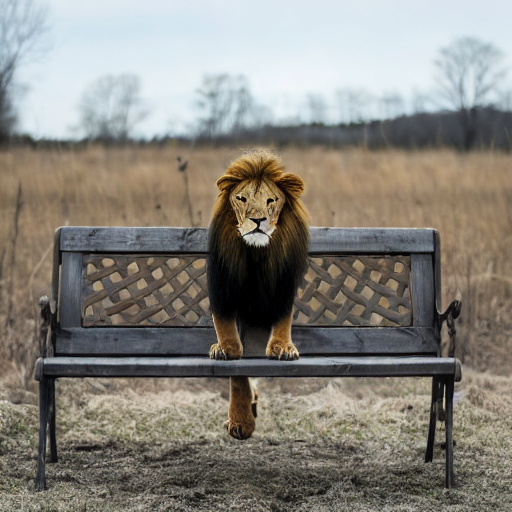
-
Stable Diffusion Inpainting API Documentation
-
API Parameters
The API POST-https://gateway.appypie.com/inpainting/v1/getImage takes the following parameters:
prompt
string, required
negative_prompt
string, optional
response_format
JavaScript Object Notation (JSON)
size
string, optional, default: 1024x1024
Integration and Implementation
To use the Stable Diffusion Inpainting API, developers must make POST requests to the specified inpainting endpoint with the appropriate headers and request body. The request body should include the Input Image, a mask indicating the regions to be modified, the text Prompt, and any optional parameters such as negative prompts, guidance_scale, and image size.
Base URL
https://gateway.appypie.com/inpainting/v1/getImage
Endpoints
POST /getImage
This endpoint generates and modifies images based on the text prompts.
Request
- URL: https://gateway.appypie.com/inpainting/v1/getImage
- Method: POST
- Headers:
- Content-Type: application/json
- Cache-Control: no-cache
- Ocp-Apim-Subscription-Key: {subscription_key}
- Body:
JSON
{ "prompt": "Change to a lion" }
- HTTP Status Codes:
- 200 OK: The request was successful, and the generated image is included in the response body.
- 400 Bad Request: The request must be corrected or include some arguments.
- 401 Unauthorized: The API key provided in the header is invalid.
- 500 Internal Server Error: An error occurred on the server while processing the request.
- Sample Response:
JSON
{ "status": 200, "content-type": "image/png", "content-length": "size_in_bytes", "image_data": "base64_encoded_image_data" }
- URL: https://gateway.appypie.com/inpainting/v1/getImageStream
- Method: POST
- Headers:
- Content-Type: application/json
- Cache-Control: no-cache
- Ocp-Apim-Subscription-Key: {subscription_key}
- Body:
JSON
{ "prompt": "Change to a lion" "System prompt": "You are supposed to be the best image generator." }
- Error Field Contract:
- code: An integer that indicates the HTTP status code (e.g., 400, 401, 500).
- message: A clear and concise description of what the error is about.
- traceId: A unique identifier that can be used to trace the request in case of an issue.
- Image Editing and Manipulation: The Stable Diffusion Inpainting API excels at editing and manipulating existing images. Users can provide an Input Image, a mask indicating the regions to be modified, and a text Prompt describing the desired changes. The API will then generate a new output image that matches the Prompt while preserving the unmasked parts of the original image. This is useful for tasks like object removal, background replacement, and image enhancement.
- Content Creation: The Inpainting API can be leveraged to quickly generate unique, high-quality images for a variety of content needs, such as blog posts, social media posts, product images, and more. By providing a Prompt describing the desired image, the API can create custom visuals tailored to the user's requirements.
- Personalized Marketing: The Stable Diffusion Inpainting API can be used to generate personalized images for marketing campaigns. Users can provide an Input Image of a product or scene, and the API can modify it based on a Prompt to create unique visuals for each customer or target audience.
- Game Asset Generation: Game developers can use the Inpainting API to generate unique game assets, such as characters, environments, and props, by providing Prompts that describe the desired assets. This can significantly reduce the time and effort required for asset creation.
- AI-Assisted Art and Design: Artists and designers can leverage the Stable Diffusion Inpainting API to enhance their creative process. By providing Input Images and Prompts, they can explore new ideas, experiment with different styles, and generate unique visual concepts more efficiently. This maximizes the desired result in AI image creation, ensuring image quality and sharp focus are at maximum value while producing blank images with the stable diffusion model.
- Guidance Scale Adjustment: The API allows users to fine-tune the guidance_scale parameter, enabling precise control over the inpainting process. This adjustment helps achieve the desired result and ensures high image quality in the output image.
- Customizable Prompts: Users can provide detailed Prompts to guide the image generation process. This feature allows for highly specific modifications, ensuring that the generated image closely aligns with the user's vision. Users can adjust the Prompt strength to influence the inpainting results more accurately.
- Negative Prompts: The API supports negative prompts, which help exclude unwanted elements from the generated image. This ensures that the output image is free from any undesired features.
- Flexible Input and Output Sizes: The Stable Diffusion Inpainting API supports various image sizes, allowing users to specify the dimensions of both the Input Image and the output image. This flexibility is crucial for different applications, from web content to high-resolution prints. The API is designed to handle high resolution images, ensuring the quality of the entire image.
- Masking Capabilities: Users can provide a mask to indicate specific regions of the Input Image that need modification. This feature ensures that only the targeted areas are altered, preserving the integrity of the rest of the image. The API effectively processes masked content to deliver precise modifications.
- API Key Security: Access to the API is secured through an API key, ensuring that only authorized users can utilize its features. This security measure protects the API from unauthorized access and misuse.
- Inpainting Endpoint: The API offers a dedicated inpainting endpoint that streamlines the process of submitting requests and receiving results. This endpoint is optimized for efficient handling of inpainting tasks.
- High-Quality Image Generation: Leveraging the stable diffusion model, the API generates high-quality images with sharp focus and clear details. This ensures professional-grade results for various applications. The base model used in the API ensures robust performance in various image restoration tasks.
- Web UI: The Stable Diffusion Inpainting API can be integrated with a stable diffusion webui, providing users with an intuitive interface for managing their inpainting tasks. This web UI simplifies the interaction with the API, making it accessible even to those with limited technical expertise.
- Machine Learning Models: The API leverages advanced machine learning models to perform inpainting tasks, ensuring accurate and efficient processing of images. These models enable the API to handle complex tasks and deliver high-quality visual content.
- Model Architecture: The API utilizes a latent diffusion model, which incorporates advanced machine learning techniques to inpaint images based on provided init_image, mask_image, and classifier-free guidance.
- Inference Speed: It offers efficient inference capabilities, handling high volumes of image processing requests swiftly to deliver real-time or near-real-time results via its REST API.
- Scalability: Designed to scale horizontally, the API can accommodate varying workloads and increased demand without compromising performance or latency. This scalability makes it a robust starting point for AI development projects.
- API Integration: Provides RESTful API endpoints for seamless integration into existing applications and workflows, supporting various programming languages and environments for leveraging artificial intelligence tools.
- Security: Ensures data security through robust API key authentication mechanisms, protecting user information and maintaining confidentiality during image processing tasks. Additionally, supports webhook API calls for real-time updates and notifications, enhancing integration capabilities in diverse application scenarios.
- Versatility: The inpainting endpoint can be used for a wide range of applications, including restoring damaged photos, removing unwanted objects from images, and more. This versatility makes it an ideal solution for organizations that need to generate high-quality images for a variety of purposes. Users can specify masked areas and other parts of the image to inpaint, unlocking new possibilities for digital art creation.
- Time-saving: With the inpainting endpoint, users can save time and resources compared to manual image editing processes. It can handle high volumes of requests, providing better results quickly and efficiently. Inference Endpoints like the Segmind Tiny-SD model ensure performance optimization, offering faster inferences while maintaining Max Height image quality. This efficiency is crucial for applications requiring cinematic lighting and rapid image generation.
- Customizability: Users can specify the exact area to be inpainted and adjust parameters such as the level of detail and style, resulting in highly customized images that meet their specific description of the desired result. This customizability extends to Text Generation and 3d-model integration, enabling ai art generator functionalities within various programming languages.
- Ease of Use: The Stable Diffusion inpainting feature is designed to easily change or incorporate new elements into existing images. Users can describe desired changes with a simple prompt and let the AI do the rest. This ease of use is enhanced through mask mode selections and intuitive text inputs, ensuring users can explore the full potential of their creative vision.
- Accessibility: Stable Diffusion inpainting is available through user-friendly web interfaces like Getimg.ai, allowing a wide range of users to leverage its capabilities without technical expertise. This accessibility democratizes digital art creation and expands artistic horizons, making sophisticated AI art generator tools accessible to diverse audiences. Overall, the Stable Diffusion Inpainting API provides a powerful and flexible solution for image editing and generation, enabling users to quickly create customized visuals while saving time and resources.
Responses
POST /Get image stream
This endpoint API generates images based on the provided prompts.
Request
Error Handling
The Stable Diffusion Inpainting API provides comprehensive error handling to ensure smooth operation. Common status codes include:
Overview of Stable Diffusion Inpainting Model
Stable Diffusion Inpainting model is a powerful text-to-image generation model developed by Stability AI. Unlike a traditional image editing model, the Stable Diffusion Inpainting model is designed to generate new images based on textual descriptions, rather than modifying existing images. The core functionality of the Stable Diffusion Inpainting model is to generate high-quality images from textual descriptions. Users can provide a text prompt, and the model will create a corresponding output image. This model is accessible through the Stable Diffusion API (SDXL API), which provides a flexible and easy-to-use interface for developers to integrate the Inpainting API into their applications.
The Stable Diffusion Inpainting model is trained on a large and diverse dataset, enabling it to generate a wide range of high-quality images across various genres and styles. By adjusting parameters like guidance_scale, users can fine-tune the model to generate images that better match their specific requirements. To use the Stable Diffusion Inpainting model, developers only need to obtain an API key and integrate the inpainting endpoint into their applications, making it a versatile and accessible tool for AI Inpainting and image generation.
Use Cases of the Stable Diffusion Inpainting API
The Stable Diffusion Inpainting API offers powerful capabilities for image manipulation, creation, and enhancement. By leveraging the advanced Stable Diffusion model, users can transform and generate high-quality images with precision. Here are some key use cases:
Advanced Features of the Stable Diffusion Inpainting API
The Stable Diffusion Inpainting API offers advanced features that enhance its versatility and effectiveness in various image manipulation and generation tasks. Here are some of its key advanced features:
Technical Specifications of the Stable Diffusion Inpainting API
What are the Benefits of Using Stable Diffusion Inpainting API?
The Stable Diffusion Inpainting API offers several key benefits:
Top APIs for Generative AI Models
Unlock the full potential of your projects with our Generative AI APIs. from video generation APIs to image creation, text generation, animation, 3D models, prompt generation, image restoration, and code generation, we offer advanced APIs for all your generative AI needs.